Every time you are playing around with web tests you think: why have I waited so long. Doesn’t matter, you have done it and that’s it what matters.
The last time I used Selenium 1 which is very often used for web tests. Selenium 1 is great, but has a few limitations. It is based around an injected Javascript library – the Selenium core. It works, but starting the browser with the Selenium core is often a little bit tricky. With every browser update you hope that it is still running or hope for the update, which needs to be included in the Maven plugin, is pushed to the Maven mirrors, is really working on all systems, …
But now there is Selenium 2 with the merge of Webdriver. It is no longer a Javascript core, it is a native browser plugin. And now you are much deeper inside the browser. It feels more stable from the first second and it works really well. With the Webdriver part of Selenium 2 there is a new API. But it isn’t very complicated, just different.
I can give you a damn short example which works really well for me and runs through our entire webpage. The great part: it takes screenshots of the complete page (not just the visible part) and stores them in the target folder.
First of all, the Maven pom:
[codesyntax lang="xml"] <pre><?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>de.viaboxx.tests</groupId> <artifactId>integrationtest</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>5.13.1</version> <scope>test</scope> </dependency> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium</artifactId> <version>2.0a6</version> </dependency> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-firefox-driver</artifactId> <version>2.0a6</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <configuration> <skip>true</skip> </configuration> <executions> <execution> <phase>integration-test</phase> <goals> <goal>test</goal> </goals> <configuration> <skip>false</skip> </configuration> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.5</source> <target>1.5</target> </configuration> </plugin> </plugins> </build> </project></pre> [/codesyntax]
And it follows the hole logic for the test placed under src/test/java/de/viaboxx/tests/SeleniumTest.java:
[codesyntax lang="java"] <pre>package de.viaboxx.tests; import org.apache.commons.io.FileUtils; import org.openqa.selenium.OutputType; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.Assert; import org.testng.annotations.Test; import java.io.File; import java.io.IOException; public class SeleniumTest { private static final String FOLDER_NAME = "target/screenshots/"; @Test public void website() throws IOException, InterruptedException { FirefoxDriver driver = new FirefoxDriver(); driver.get("http://www.viaboxxsystems.de"); Assert.assertEquals(driver.findElementByXPath("//h2").getText(), "Innovation"); FileUtils.copyFile(driver.getScreenshotAs(OutputType.FILE), new File(FOLDER_NAME + "startpage.png")); driver.findElementByLinkText("Team").click(); Assert.assertEquals(driver.findElementByXPath("//h2").getText(), "Team"); FileUtils.copyFile(driver.getScreenshotAs(OutputType.FILE), new File(FOLDER_NAME + "team.png")); driver.findElementByLinkText("Blog").click(); FileUtils.copyFile(driver.getScreenshotAs(OutputType.FILE), new File(FOLDER_NAME + "blog.png")); driver.findElementByLinkText("Impressum").click(); FileUtils.copyFile(driver.getScreenshotAs(OutputType.FILE), new File(FOLDER_NAME + "impressum.png")); driver.close(); } }</pre> [/codesyntax]
Run it with:
mvn integration-test
Readable, isn’t it. And that’s all – a complete working infrastructure for your web tests. The example works with Firefox, but you can also run headless with HtmlUnit, or make your tests running in IE or Chrome. The great thing: just put the application in your continuous integration server and run it on your defined build agent.
After just one evening I fall in love with the new Selenium 2. Why? Because it works much more stable in kind of browser starting and running. I think it is a great way to go.
Watch the screenshots, just taken from the target folder:
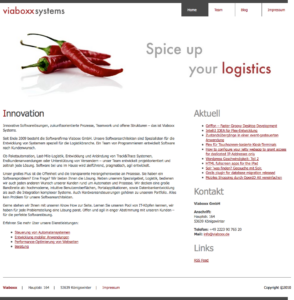
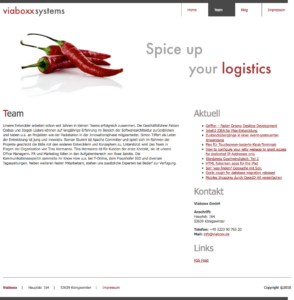
More documentation: