Imagine, that your requirement is to use a DLL, that contains .NET components under Windows. Your first thought could be JNI, but calling .NET components requires much more glue code, than just calling the functions that the DLL exports. In our projects, we have made good experiences with a framework, that uses reflection to generate proxy classes for seamless interoperation between java and .NET.
This is what I want to demonstrate in this blog post. The framework is jni4net – a bridge between java and .NET on Microsoft Windows platforms. It’s an open-source framework with an LGPL runtime license.
We just wanted to call .NET components from our Java application. It is also possible to launch a JVM and call Java from a .NET application, but this is not demonstrated in this post.
Here is our example model, the names are just arbitrary examples for a project structure with its dependencies:
- jModel.jar: some Java interfaces and models
- netLogic.DLL: .NET Code (here: Visual Basic.NET, but the same works with C# or other .NET languages as well) that use the models, implement the interfaces from jModel.jar
- jApplication.jar: A Java application using the classes from both netLogic.DLL and jModel.jar
Example Model
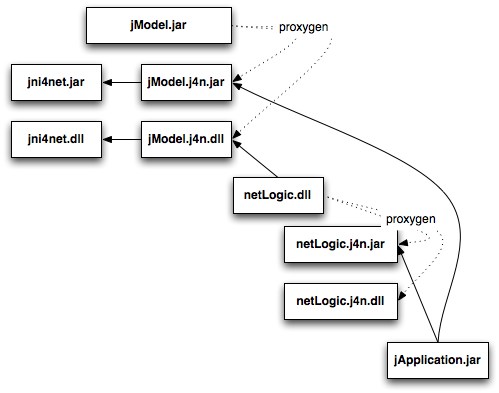
All you need is an installation of .NET (comes with Visual Studio or the Windows SDK for Server) and the jni4net framework, and, of course, a JDK for compiling the Java parts. All running under Windows.
- netLogic.dll references: jni4net.n.w32.v20-0.8.2.0.dll, jni4net.n-0.8.2.0.dll and jModel.j4n.dll
You can create a Visual Studio project for the .NET code of netLogic with those references. - jApplication.jar referenes: jModel.jar, jModel.j4n.jar and netLogic.j4n.jar
You can use Apache Ant or Maven to build it. In this example, we use Ant, because the generated artifacts of the jni4net framework can used more easily with ant, while you should upload them in a maven repository when using maven.
Build process
- Compile and create jModel.jar. Nothing special here. Just plain java. You can use ant or maven.
- Call the jni4net tool “proxygen.exe” to create jModel.j4n.jar and jModel.j4n.dll for interoperation with .NET.
The tool “proxygen.exe” also creates a shell script “build.cmd” to compile the generated code to build the .jar and .dll. - Call msbuild.exe or use the Visual Studio IDE to build the .NET project, that uses the Java classes from jModel.jar.
- Call jni4net tool “proxygen.exe” to create netLogic.j4n.jar and netLogic.j4n.dll for interoperation with Java.
- Compile and create jApplication.jar, you can use ant or maven. Don’t forget the dependencies to jModel and netLogic.j4n.dll and the jni4net.jar. (jni4net.jar/.dll are runtime components that you also need to deploy with your application)
# step 2 proxygen jModel.jar -wd target1 target1build.cmd # step 3 msbuild.exe netLogic.sln /t:Rebuild /p:Configuration=Release # step 4 proxygen.exe netLogic/obj/Release/netLogic.dll -wd target2 -dp target1jModel.j4n.dll -cp target1jModel.j4n.jar;target1jModel.jar target2build.cmd
Bootstrap: Java calling VB.NET
Java: Bridge.init(); Bridge.LoadAndRegisterAssemblyFrom(new File("jModel.j4n.dll")); Bridge.LoadAndRegisterAssemblyFrom(new File("netLogic.j4n.dll"));
Data Type Conversion between Java and .NET
The bridge automatically converts most data types to their counterpart.
Java: public interface ExampleModule { String[] getTypes(); } VB.NET: Public Class MyVBExampleModule Implements ExampleModule Public Function getTypes() As java.lang.String() Implements ExampleModule.getTypes ... End Function End Class
For more information, refer to the jni4net homepage. Although the framework claims to have “alpha quality”, there are no problems when using it in some of our products.
We hope that the barrier to leave the Java world, through these examples, is diminished!